Introduction
Nativo
is an enhanced version of the WETH contract, which provides a way to wrap
the native cryptocurrency of any supported EVM network into an ERC20 token, thus enabling more
sophisticated interaction with smart contracts and DApps on various blockchains.
Wrapped Ether (WETH) has been an indispensable resource in the Ethereum ecosystem, allowing Ether (ETH) to interface with Ethereum-based protocols through the ERC20 standard. Despite its importance, however, WETH has begun to show its age. It lacks several features that could make Ethereum-based protocols run more smoothly, and was deployed bypassing Solidity's optimizer, resulting in suboptimal gas usage and slower operation. Nativo arises to address these limitations, offering an updated and improved version of WETH with advanced features and a more efficient operation in terms of gas.
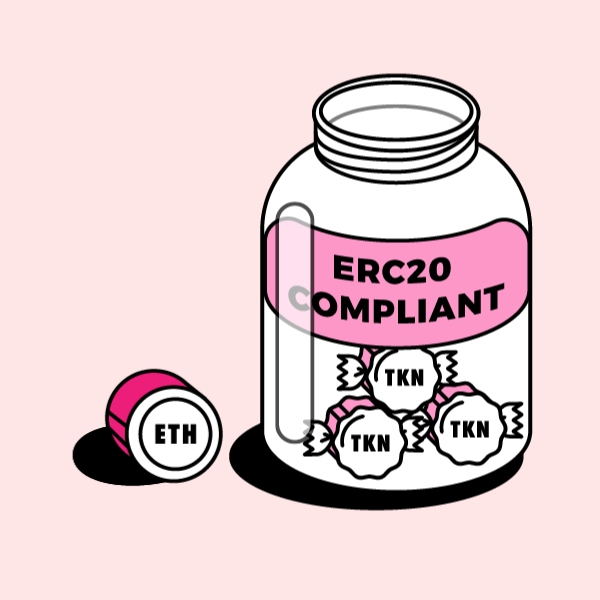
But Why Can't We Just Use ETH?
One challenge I like to posit to our students is “If you wanted to go directly to the ”ETH Contract" to manipulate ETH in Etherscan, where would you go?" This contract of course does not exist, and everything ETH can do is built into the core code of Ethereum itself. ETH is limited in what it can do. You can Transfer it, use it to pay for gas, and add it as a cost for a method (think Mint, for example). Beyond that, you can't do anything too complicated with it.
Get started
To get some Nativo
tokens, you can wrap your ETH using the deposit
function via this
web interface
If you are using a smart contract you can use this interface: INativo.sol.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.4;
interface Nativo {
error AddressZero();
error AllowanceOverflow();
error AllowanceUnderflow();
error ERC3156ExceededMaxLoan(uint256 maxLoan);
error ERC3156InvalidReceiver(address receiver);
error ERC3156UnsupportedToken(address token);
error InsufficientAllowance();
error InsufficientBalance();
error InvalidPermit();
error PermitExpired();
error WithdrawFailed();
event Approval(
address indexed owner,
address indexed spender,
uint256 amount
);
event RecoverNativo(address indexed account, uint256 amount);
event Transfer(address indexed fxrom, address indexed to, uint256 amount);
fallback() external payable;
function DOMAIN_SEPARATOR() external view returns (bytes32);
function allowance(address user, address spender)
external
view
returns (uint256 amount);
function approve(address spender, uint256 amount) external returns (bool);
function approveAndCall(address spender, uint256 amount)
external
returns (bool);
function approveAndCall(
address spender,
uint256 amount,
bytes memory data
) external returns (bool);
function balanceOf(address account)
external
view
returns (uint256 _balance);
function decimals() external view returns (uint8);
function deposit() external payable;
function depositTo(address to) external payable;
function flashFee(address token, uint256 amount)
external
view
returns (uint256);
function flashLoan(
address receiver,
address token,
uint256 amount,
bytes memory data
) external returns (bool);
function maxFlashLoan(address token)
external
view
returns (uint256 maxLoan);
function name() external view returns (string memory);
function nonces(address user) external view returns (uint256 nonce);
function permit(
address owner,
address spender,
uint256 value,
uint256 deadline,
uint8 v,
bytes32 r,
bytes32 s
) external;
function recoverERC20(address token, uint256 amount) external;
function recoverNativo(address account) external;
function supportsInterface(bytes4 interfaceId)
external
view
returns (bool result);
function symbol() external view returns (string memory);
function totalSupply() external view returns (uint256 totalSupply_);
function transfer(address to, uint256 amount) external returns (bool);
function transferAndCall(address to, uint256 amount)
external
returns (bool);
function transferAndCall(
address to,
uint256 amount,
bytes memory data
) external returns (bool);
function transferFrom(
address from,
address to,
uint256 amount
) external returns (bool);
function transferFromAndCall(
address from,
address to,
uint256 amount,
bytes memory data
) external returns (bool);
function transferFromAndCall(
address from,
address to,
uint256 amount
) external returns (bool);
function treasury() external view returns (address);
function withdraw(uint256 amount) external;
function withdrawFromTo(
address from,
address to,
uint256 amount
) external;
function withdrawTo(address to, uint256 amount) external;
receive() external payable;
}